How to Translate Excel Documents using Python and ChatGPT: A Step-by-Step Guide
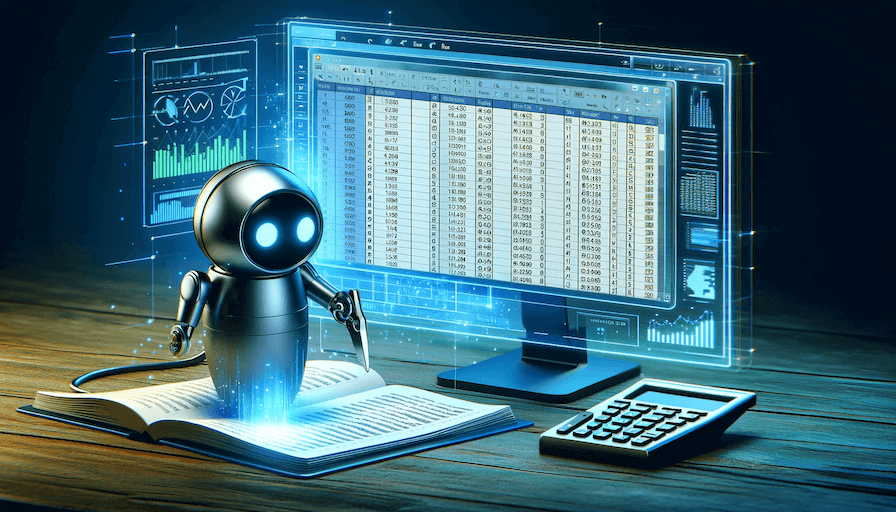
Introduction
There are many translation tools out there. But not all of them give good results. This is why we like ChatGPT so much.
ChatGPT is made by OpenAI. It's more than just a normal tool. It's very good at many tasks, especially translating. Often, its translations are as good as those done by professionals.
By the way, you can use our service for easy translations. Just upload your Excel file. Our site will translate it for you. After it's done, download your file. The format stays the same.
But, how can we use ChatGPT and Python to translate Excel files on our own? In this blog, we'll show you how to do it step by step. We want to make sure your translations are quick and top quality.
Prerequisites
Before we start, there are a few things you need:
- Basic Python Knowledge This article will have some coding. It's good if you know some basic Python. But don't worry, we'll explain everything.
- Python Make sure you have Python installed on your computer. If not, you can download it from the official website.
- pip This is a tool that helps you install Python libraries. If you've installed Python3, you likely already have pip.
- OpenAI's GPT-4 API Key To use ChatGPT for translation, you need this key. If you don't have one, visit OpenAI's website to get it.
- Your Excel File Have the Excel file you want to translate ready.
Peeking Inside an Excel File
When you save a spreadsheet in Excel with the .xlsx extension, what you're really doing is packaging up a bunch of files into one. This file format is known as OpenXML. If you've ever been curious about the inner workings of an Excel file, let's unzip it and take a look:
1. Worksheets Folder (xl/worksheets/):
Inside this folder, you'll find an XML file for each worksheet in your Excel file. So, if you had three tabs or sheets in your workbook, you'll find sheet1.xml, sheet2.xml, and sheet3.xml here.
Each of these XML files represents the rows, columns, and cells of its respective sheet. It's where your actual data lives.
2. Styles (xl/styles.xml):
This XML file is like the fashion designer for your Excel data. It contains the definitions for all the styles used in your workbook.
If you've ever wondered how Excel remembers which cells are blue, which texts are bold, or which numbers are formatted as currency, it's all defined here.
3. Shared Strings (xl/sharedStrings.xml):
To save space and make the file more efficient, Excel places all the unique strings (texts) used across your worksheets into this file.
For instance, if you had the word "Total" repeated 1,000 times across your workbook, it's stored just once here, and then referenced everywhere it's used.
4. Workbook Structure (xl/workbook.xml):
Think of this as the table of contents for your Excel file.
It outlines the structure of the workbook, detailing things like which sheets are present, their order, and even some properties like sheet protection.
When working with tools like openpyxl, these details are mostly hidden from you, allowing you to work with Excel data in a more intuitive way. However, understanding this structure can provide insights, especially if you're looking to do advanced operations or troubleshoot issues.
Reading and Writing Excel Documents with openpyxl
openpyxl is a Python library made especially for reading and writing Excel files (both .xlsx and .xlsm). It allows you to work directly with Excel sheets and cells.
Here's how to get started:
1. Install openpyxl
If you haven't done so yet, install openpyxl using pip:
pip install openpyxl
2. Excel Basics with openpyxl
To work effectively with openpyxl, it's beneficial to understand some core concepts of Excel and how they translate into openpyxl operations:
A. Workbook: When we talk about an Excel file, we're referring to a Workbook. In openpyxl, you can think of a workbook as the main file you're working with.
from openpyxl import load_workbook
# Loading an existing workbook
workbook = load_workbook(filename="sample.xlsx")
B. Sheet: A workbook contains individual sheets, which are like pages in a book. Each sheet holds your data in a table format. With openpyxl, you can access the active sheet or any sheet by its name.
# Getting the active sheet
sheet = workbook.active
# Accessing a sheet by name
another_sheet = workbook["Sheet2"]
C. Cell: Sheets are made of rows and columns. Where a row and column intersect, you have a cell. This is where your data resides. openpyxl allows you to read and write data from and to these cells.
# Reading the value from cell A1
cell_value = sheet["A1"].value
# Writing to cell B1
sheet["B1"] = "Hello, Excel!"
D. Row and Column: In Excel, rows run horizontally and are numbered, while columns run vertically and are labeled with letters. openpyxl provides intuitive methods to iterate through them.
# Iterating through rows
for row in sheet.iter_rows(values_only=True):
for value in row:
print(value)
3. Reading and Editing the Excel File
from openpyxl import load_workbook
# Load the workbook and select the active sheet
workbook = load_workbook(filename='your_file.xlsx')
# Loop through all sheets
for sheet in workbook:
# Loop through all rows and columns in the sheet
for row in sheet.iter_rows():
for cell in row:
original_text = cell.value
# Check if the cell contains text
if isinstance(original_text, str):
# Replace with your ChatGPT translation method
translated_text = TRANSLATION_METHOD_HERE(original_text)
cell.value = translated_text
# Save the changes to the same file
workbook.save('your_file.xlsx')
In the code above, replace "TRANSLATION_METHOD_HERE" with the method you'll use to translate text using ChatGPT. This approach will go through every cell in the workbook. If a cell contains text, it will be translated.
Translating with ChatGPT
After loading your Excel data with openpyxl, the next step is translating the content using ChatGPT. Here's a step-by-step guide:
1. Install the OpenAI Python client:
This client allows us to interact with ChatGPT and obtain translations.
pip install openai
2. Set Up ChatGPT Translation:
import openai
# Initialize the OpenAI API with your key
# openai.api_key = 'YOUR_OPENAI_API_KEY'
def translate_text(text, target="en"):
content = "Translate the following text to " + target + ": " + text
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": content}]
)
return response.choices[0].message.content
Of course, if you prefer, you can easily swap out ChatGPT with DeepL or another translation service. The beauty of the translate function is its simplicity, allowing for flexible integration with various translation tools.
3. Integrate Translation with Excel Iteration:
Now, merge this translation function with the previous openpyxl code. For each cell containing text, call translate_text to get its translation.
# ... (Your openpyxl code to read the Excel file)
for row in sheet.iter_rows():
for cell in row:
original_text = cell.value
if isinstance(original_text, str):
# Replace 'TARGET' with desired language code
translated_text = translate_text(original_text, "TARGET")
cell.value = translated_text
workbook.save('your_translated_file.xlsx')
4. Remember your API Costs:
Note that translating large Excel files might lead to a significant number of API calls, which could be costly. Always monitor your usage to avoid unexpected charges.
Complete Code: Excel Translation to French with ChatGPT and openpyxl
With this code, you can quickly translate English text in an Excel file to French. We'll use the power of ChatGPT for translation and openpyxl to handle the Excel file. Let's get started!
from openpyxl import load_workbook
import openai
# Initialize the OpenAI API with your key
openai.api_key = 'YOUR_OPENAI_API_KEY'
def translate_text(text, target="fr"):
content = "Translate the following text to " + target + ": " + text
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[{"role": "user", "content": content}]
)
return response.choices[0].message.content
# Load the workbook and select the active sheet
workbook = load_workbook(filename='your_file.xlsx')
# Loop through all sheets
for sheet in workbook:
# Loop through all rows and columns in the sheet
for row in sheet.iter_rows():
for cell in row:
original_text = cell.value
# Check if the cell contains text
if isinstance(original_text, str):
translated_text = translate_text(original_text)
cell.value = translated_text
# Save the changes to the same file
workbook.save('your_translated_file.xlsx')
Conclusion
Using ChatGPT and openpyxl, we've crafted a simple tool to translate English text in Excel to languages like French, Japanese and German. While our example showcased French, you can adapt this method for any language supported by ChatGPT. The entire process is impressively straightforward, requiring just around 30 lines of code.
Also, you can use our website for easy translations. Just upload your Excel, and we'll translate it for you. After it's done, download your file. Simple and quick!
Frequently Asked Questions (FAQs) and Solutions
What if I get an error related to the API limit?
Answer: The OpenAI platform has certain rate limits depending on your account type. If you encounter a rate limit error, you may need to add some delay in your script between translation calls or consider upgrading your OpenAI account to a higher tier.
How can I translate into multiple languages simultaneously?
Answer: You can modify the code to loop through a list of target languages. For each cell, you can call the translation function multiple times for each language and save the results to separate Excel sheets or files.
Is there a cost associated with using ChatGPT for translation?
Answer: Yes, using the OpenAI API may come with associated costs, depending on your usage and subscription plan. Always keep an eye on your usage to avoid unexpected bills.
This article primarily discusses how to translate Excel files. If you wish to use Python and ChatGPT to translate Word documents, you can refer to Automating Word Document Translation with Python and ChatGPT.