Automating Word Document Translation with Python and ChatGPT
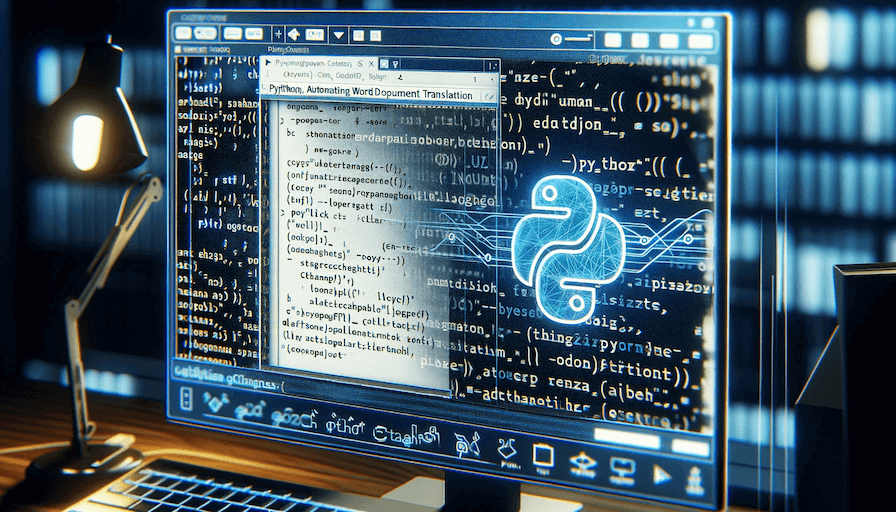
Introduction
In this guide, we're diving into a simple way to turn an entire Word file into another language using Python and ChatGPT. Think of it like having a super-smart translator buddy, thanks to OpenAI's ChatGPT.
For readers who have experienced the challenges of translating Excel documents, I've previously written a guide titled How to Translate Excel Documents using Python and ChatGPT: A Step-by-Step Guide , which provides a step-by-step approach tailored specifically to Excel files.
If you are looking for an easier alternative, check out doc2lang.com which can translate Excel and Word files with just a simple upload.
Understanding the DOCX Format
When we talk about "Word files", we're typically referring to files with a .docx extension. This format, introduced with Microsoft Word 2007, has since become the standard for Word documents. But what's inside a .docx file isn't just plain text; it's a combination of XML structures, media, styles, and more, all packed together. Here's a simple breakdown:
- XML-Based: Unlike the older .doc format, which was a binary file, .docx is based on XML (Extensible Markup Language). This makes it more accessible and interoperable.
- ZIP Container: If you've ever tried renaming a .docx file to .zip and extracting it, you'd see a bunch of folders and files. This is because a .docx file is essentially a zipped collection of various resources.
- Contained Components: Inside the ZIP container, you'll find:
- document.xml: This contains the main content of the document.
- styles.xml: Contains the styles used throughout the document.
- Media folder: Any images or media included in the document.
- And more, including theme, font, and settings information.
- Styles and Formatting: One of the reasons Word files can look so diverse and beautiful is because of the myriad of styles and formatting options available. These styles dictate how headers, paragraphs, links, and other elements appear.
By understanding the structure and components of a .docx file, we can better navigate and manipulate its contents, making the translation process more efficient.
Setting Up ChatGPT for Translation
If you've delved into our previous guide on translating Excel documents, the process for setting up ChatGPT should be familiar. For newcomers, getting ChatGPT ready for translation is a breeze.
A brief recap:
-
Install the OpenAI Python client:
pip install openai
-
Initialize and set up the translation function:
import openai # Initialize the OpenAI API with your key openai.api_key = 'YOUR_OPENAI_API_KEY' def translate_text(text): content = "Translate the following English text to Spanish: " + text response = openai.ChatCompletion.create( model="gpt-4", messages=[{"role": "user", "content": content}] ) return response.choices[0].message.content
For those seeking a deeper dive, do check out our comprehensive article.
The previous example translated from English to French, whereas this example demonstrates translation from English into Spanish. Of course, you can change the source or target language to any language. For example, translating from German into Arabic, or from Spanish into Japanese.
The Translation Process
Translating an entire Word file involves several steps, especially when considering the complexity of .docx files. These files can contain not just text, but images, tables, headers, footers, and more. Here's a straightforward process to achieve accurate translations:
-
Extract Text from the Word File:
- Before any translation can occur, the textual content within the Word file needs to be extracted.
- Python's python-docx library is perfect for this. Install it using:
pip install python-docx
- Extract the text with:
from docx import Document doc = Document('path_to_your_file.docx') full_text = [para.text for para in doc.paragraphs]
-
Chunking the Text:
- Language models like ChatGPT have token limits. Ensure that you break the extracted text into manageable chunks.
- This step is crucial for maintaining context and not truncating sentences.
-
Translate Each Chunk with ChatGPT:
- Use the translate_text function set up earlier to translate each chunk.
- Iteratively go through each chunk and store the translated text.
translated_chunks = [translate_text(chunk) for chunk in text_chunks]
-
Reconstruct the Word File:
- After translation, the content should be placed back into a Word file, preserving the original format.
- Using python-docx, create a new document and insert the translated content.
translated_doc = Document() for chunk in translated_chunks: translated_doc.add_paragraph(chunk) translated_doc.save('translated_file.docx')
-
Post-Translation Review:
- No translation process is perfect. It's recommended to manually review the translated document.
- Check for any issues, such as incorrect translations, formatting errors, or missing content.
By following this process, you'll be able to effectively leverage ChatGPT's capabilities and Python's versatility to produce high-quality translations of Word documents.
Conclusion
Translating Word documents can present unique challenges due to the varied content types and rich formatting options they offer. However, with the powerful combination of Python and ChatGPT, we have shown that these challenges can be tackled effectively. This guide offers a foundation for automating translation tasks, ensuring consistency, and saving valuable time. As always, it's crucial to review translations, especially for professional or official documents, to ensure the utmost accuracy. The fusion of automated tools and human expertise will always yield the best results. Happy translating!